Building sophisticated applications powered by Large Language Models (LLMs) can be a complex task, especially when it comes to managing memory and context effectively. The Haystack memory system for LLM applications provides an open-source solution to this challenge, enabling developers to create powerful workflows with ease. Designed with a modular pipeline architecture, Haystack simplifies data retrieval, preprocessing, and response generation, making it an essential tool for building intelligent, context-aware AI systems.
With its focus on Retrieval-Augmented Generation (RAG) and seamless integration with various components, Haystack empowers developers to enhance LLM capabilities, unlocking innovative applications across industries. In this article, we’ll explore Haystack’s features, architecture, and practical use cases, along with step-by-step examples to help you get started.
What is Haystack?
Haystack is a Python framework designed to simplify the development of custom applications powered by LLMs. It provides a modular pipeline architecture, allowing developers to seamlessly chain components and build workflows tailored to their needs.
Core Concepts
- Pipelines: Define a sequence of operations for processing and generating information, enabling highly customizable workflows.
- Components: Haystack offers a variety of components for tasks such as data retrieval, text embedding, and response generation.
- RAG (Retrieval-Augmented Generation): Haystack combines retrieval mechanisms with generative capabilities of LLMs for accurate, context-aware applications.
Key Features of Haystack
1. Modular Pipeline Architecture
Haystack’s pipeline system allows developers to:
- Use predefined pipelines for common tasks like question answering and indexing.
- Create custom pipelines by chaining components such as retrievers, preprocessors, and generators.
2. Rich Library of Components
Haystack includes components for:
- Fetchers: Retrieve data from web pages or local files.
- Converters: Transform raw data into usable formats.
- Preprocessors: Clean and segment text for optimal model performance.
- Embedders: Generate semantic vector embeddings.
- Retrievers: Fetch the most relevant information for user queries.
- Generators: Generate text responses using LLMs.
- Writers: Store processed data for later use.
3. Retrieval-Augmented Generation (RAG)
Haystack excels in RAG, where LLMs combine generative abilities with a retrieval mechanism to:
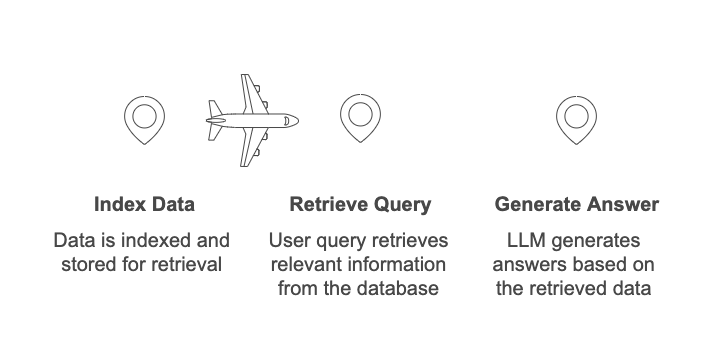
- Access relevant knowledge bases.
- Provide precise, context-aware answers.
- Maintain session continuity for user interactions.
Getting Started with Haystack
Installation
To get started, install Haystack via pip:
pip install haystack-ai
Environment Setup
Set API keys for components that use external APIs, like OpenAI:
export OPENAI_API_KEY="your_openai_api_key"
Examples of Haystack in Action
1. Question Answering on a Webpage
Objective: Build a pipeline to answer questions about a webpage’s content.
from haystack.nodes import LinkContentFetcher, HTMLToDocument, OpenAIGenerator
from haystack.pipelines import Pipeline
# Define the pipeline
pipeline = Pipeline()
pipeline.add_node(component=LinkContentFetcher(), name="LinkFetcher", inputs=["Query"])
pipeline.add_node(component=HTMLToDocument(), name="HTMLConverter", inputs=["LinkFetcher"])
pipeline.add_node(component=OpenAIGenerator(), name="Generator", inputs=["HTMLConverter"])
# Run the pipeline
result = pipeline.run(query="What is Haystack?", params={"url": "https://example.com"})
print(result)
2. Retrieval Augmented Generation (RAG) with a Text Document
Objective: Build a RAG pipeline to answer questions based on a document.
Indexing Pipeline
from haystack.nodes import TextFileToDocument, DocumentCleaner, DocumentSplitter, OpenAIDocumentEmbedder, DocumentWriter
from haystack.pipelines import Pipeline
# Define the indexing pipeline
indexing_pipeline = Pipeline()
indexing_pipeline.add_node(component=TextFileToDocument(), name="FileConverter", inputs=["File"])
indexing_pipeline.add_node(component=DocumentCleaner(), name="Cleaner", inputs=["FileConverter"])
indexing_pipeline.add_node(component=DocumentSplitter(), name="Splitter", inputs=["Cleaner"])
indexing_pipeline.add_node(component=OpenAIDocumentEmbedder(), name="Embedder", inputs=["Splitter"])
indexing_pipeline.add_node(component=DocumentWriter(), name="Writer", inputs=["Embedder"])
# Index a document
indexing_pipeline.run(file_path="sample.txt")
RAG Pipeline
from haystack.nodes import InMemoryEmbeddingRetriever, PromptBuilder, OpenAIGenerator
from haystack.pipelines import Pipeline
# Define the RAG pipeline
rag_pipeline = Pipeline()
rag_pipeline.add_node(component=InMemoryEmbeddingRetriever(), name="Retriever", inputs=["Query"])
rag_pipeline.add_node(component=PromptBuilder(), name="PromptBuilder", inputs=["Retriever"])
rag_pipeline.add_node(component=OpenAIGenerator(), name="Generator", inputs=["PromptBuilder"])
# Query the RAG pipeline
result = rag_pipeline.run(query="Explain the content of the document.")
print(result)
Why Choose Haystack?
- Flexibility: Modular architecture allows for tailored solutions.
- Ease of Use: Predefined pipelines simplify development.
- Scalability: Handle complex workflows and interactions efficiently.
- Open Source: Active community support and regular updates.
Suggestions for Further Exploration
- Custom Pipelines: Experiment with different combinations of components to create unique workflows.
- Advanced RAG Applications: Explore how RAG pipelines can enhance knowledge-intensive domains like healthcare and legal services.
- Integrations: Combine Haystack with tools like LangChain or knowledge graphs for enriched applications.
Conclusion
Haystack empowers developers to build sophisticated and scalable applications leveraging the power of LLMs. Its modular design, rich library of components, and support for RAG make it an indispensable tool for developers looking to create intelligent, context-aware systems.
With comprehensive documentation, active community support, and predefined templates, Haystack provides a strong foundation for building next-generation AI solutions. Dive into Haystack today and unlock the potential of LLMs for your projects.
Further Reading
Explore More
- AI Services: Explore our AI services for more details.
- Digital Product Development: Discover our digital product development expertise.
- Design Innovation: Learn about our design innovation approach.
Leave a Reply